Usage
Progress indicators are visual displays that help users keep track of their progress during a specific task, process flow or steps in a phased base process. Progress indicators communicate how many steps are needed to complete a process. They are used in a variety of contexts including online sign up, a multi-step form, and onboarding. They manage expectations upfront and let users know how much time and effort a process will take.
In Jutro, progress indicators come in different layouts: steps, progress bar and phases.
The steps layout shows the number of steps in a process the user must go through to complete a task. For example, to complete a profile a user must provide information like qualifications, home details etc. These can be set as steps that are required before a task is complete.
The progress bar shows the current progress status of a system operation like loading, uploading, submitting etc. It also shows the progress of a particular task before completion.
The phases/steps layout shows the progress of users completing a task broken down into phases or steps.
Anatomy
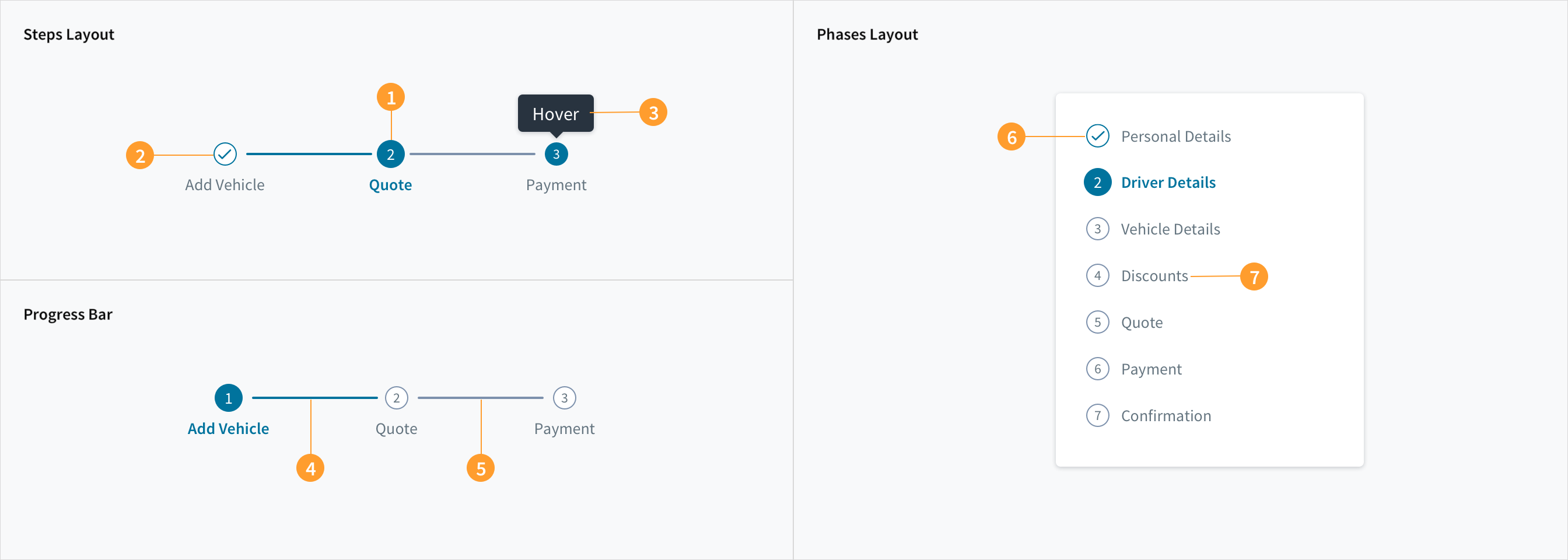
Steps layout
- Step number: Serves as a label for the current step in a task/process flow.
- Checkmark icon: lets the user know that a step is complete. The checkmark replaces the step number after completion.
- Tool tip: provides context of a step upon hover. It shows the full label of the hovered step.
Progress bar
- Fill: The fill moves as the user completes a task. When a task is complete the fill covers the entire bar.
- Bar: The bar is a visual representation of the entire task process.
Phases layout
- Icon: The icon is a visual representation of the label in a phase.
- Label: The label establishes context for a particular phase, e.g., "Payment."
Best practices
- Ensure the steps layouts are displayed in a logical order, from start to end (left to right).
- Users should be able to return to previous steps for review and to update details.
- Use icons that relate to their labels.
- Visually display the current step the user is on.
- Provide visual indication that a previous step/phase is complete.
- Ensure the steps are parsed into logical groupings, simple and clear to support understanding.
- Make sure all steps are visible.
- Keep the steps to a minimum of 3 and a maximum of 7.
Interactions
Progress indicators have enabled, disabled, active and hover states.
- Enabled: communicates to the user that the element is enabled for interaction. By default, the first step/current step in the step layout is enabled.
- Disabled: communicates to the user that the element is not interactive. Next phases/steps are disabled until the previous step/phase is complete. The steps are grayed out.
- Hover: indicates that the user has placed a cursor over the element (desktop only). When users hover over a step icon, a tooltip appears with the label, establishing context for a particular step. In this state the cursor changes to finger pointer.
- Active: indicates that the user is clicking or tapping the element. An active step/phase is highlighted.
UX writing considerations
- Keep labels short and clearly identify the step.
- Labels use sentence case, i.e. capitalize the first word of a phrase and any proper nouns.
- When using tooltips in conjunction with a step progress bar, tooltip content should only contain one or two words.
Accessibility
The Jutro Progress indicators provide information about the status of an ongoing process, such as submitting a form or setting up a quote. There are three types of Progress indicators and they each take the form of a horizontal bar.
- Keyboard interaction: Each active step can be focused on via the keyboard.
- Color: The contrast ratio of textual elements against their background is above 4.5:1 as per WCAG 2.0 AA requirements.
- Zoom: All content is visible and functional up to & including 200% zoom.
In addition to the above accessibility concerns, the following aspects have been implemented with respect to screen reader interaction:
Phase progress bar
The Phrase progress bar represents an entire process. It contains a series of smaller independent progress bars that represent phases in that process. The component implements the HTML5 landmark <nav>
tag and each individual phrase is identified by it's name (via an aria-label
value) and the WAI-ARIA role='link'
Simple progress bar
The Simple progress bar includes the WAI-ARIA role of 'progressbar'. It also includes the WAI-ARIA attributes, aria-valuetext
, aria-valuenow
, aria-valuemin
and aria-valuemax
to assist in providing additional meaning to assistive technologies.
Step progress bar
The Step progress bar comprises a series of steps to communicate completion status. It utilizes the HTML5 landmark <nav>
tag and each step in the process is assigned the WAI-ARIA role of 'link'. The visual textual value is also included within an aria-label
attribute.
These components have been validated to meet the WCAG 2.0 AA accessibility guidelines. However, changes made by the content author can affect accessibility conformance.
- Ensure that if using links, each step directs the user to the correct destination.
Code
Simple progress bar
The SimpleProgressBar
shows a simple bar to illustrate progress.
Adding steps and labels to simple progress bar
SimpleProgressBar
requires two props to work:
completed
- the number of steps already completed
total
- total number of steps
For example:
<SimpleProgressBar
completed={2}
total={10}
/>
If showProgressLabel
prop is set to true
, you can provide a label
which is displayed as the title of the progress bar. By default, the label
appears below the the progress bar. You can set labelPosition
to display it above the progress bar.
By default, steps are displayed as fractions, for example 2/10
. If showPercentageProgress
is set to true
, steps are displayed as a percentage (e.g., 20%
).
<SimpleProgressBar
completed={2}
total={10}
showPercentageProgress
showProgressLabel
label={'Progress bar example'}
labelPosition={'bottom'}
/>
Here's the presentation metadata for this example:
{
"$schema": "../metadata.schema.json",
"jutro": "10.3.6",
"simple.progress": {
"component": "SimpleProgressBar",
"id": "simpleProgressId",
"type": "element",
"componentProps": {
"completed": "2",
"total": "10",
"showPercentageProgress": true,
"showProgressLabel": true,
"label": "Progress bar example",
"labelPosition": "bottom"
}
}
}
You can use the path
prop in metadata to connect the completed
prop to certain values in metadata.
Here's the presentation metadata for this example:
{
"$schema": "../metadata.schema.json",
"jutro": "10.3.6",
"hooked.simple.progress.bar": {
"id": "wrapper",
"type": "container",
"component": "Card",
"content": [
{
"component": "SimpleProgressBar",
"id": "progressBar",
"type": "element",
"componentProps": {
"path": "baseData.step",
"label": "Progress bar",
"total": "10",
"showPercentageProgress": true
}
},
{
"id": "progressBarControl",
"type": "field",
"datatype": "number",
"componentProps": {
"path": "baseData.step",
"label": {
"id": "example.page.stepControl",
"defaultMessage": "Progress Bar Control"
},
"minValue": 0,
"maxValue": 10,
"defaultValue": "3"
}
}
]
}
}
In this example, we use the path
prop to map the progress bar and a number input to the same number. That way, the number set in the number field becomes the number of completed
steps in the progress bar.
This is a simple example, but with some additional code we can pass any value as completed
. You can see working example in Examples\Metadata-driven Example
in Storybook.
Connecting simple progress bar to steps in code (Wizard example)
We can set the completed
prop on render to achieve different goals. Here is an example of how to use a simple progress bar in the Wizard Next component.
const { pathname } = location;
const stepRoutes = steps.map(step => step?.route);
const currentStep = stepRoutes.indexOf(pathname.replace(`${path}/`, ''));
const renderSimpleProgressBar = () => (
<SimpleProgressBar
completed={currentStep}
total={steps.length}
showProgressLabel={false}
/>
);
return (
<WizardExampleContext.Provider value={exampleContext}>
<Wizard
steps={steps}
renderProgressBar={renderSimpleProgressBar}
{..otherProps}
/>
</WizardExampleContext.Provider>
);
Wizard Next has a prop to render a custom progress bar. We set total
to the length of the steps in the Wizard. We also calculate currentStep
using the current location in the wizard.
Phase progress bar
The PhaseProgressBar
shows progress divided into distinct phases.
Adding steps to phase progress bar
To render progress steps, pass an array of objects to the phases
prop. phases
is a required prop.
Each phase needs a title
and steps
.
const phases = [
{
title: 'Your Business',
icon: 'gw-home-work',
steps: [{ title: 'Your Information' }, { title: 'Qualification' }],
},
{
title: 'Quotes',
icon: 'gw-user-info',
steps: [{ title: 'Your Home' }],
},
{
title: 'Final Details',
icon: 'gw-insert-drive-file',
steps: [{ title: 'Details' }, { title: 'More details' }],
},
{
title: 'Payment',
icon: 'gw-credit-card',
steps: [
{ title: 'Construction' },
{ title: 'Your Discounts' },
{ title: 'Your Quote' },
],
},
];
<PhaseProgressBar phases={phases} />;
Here's the presentation metadata for this example:
{
"$schema": "../metadata.schema.json",
"jutro": "10.3.6",
"phase.progress.example": {
"component": "PhaseProgressBar",
"id": "phaseProgressId",
"type": "element",
"componentProps": {
"phases": [
{
"title": "Your Business",
"icon": "gw-home-work",
"steps": [
{
"title": "Your Information",
"visited": true
},
{
"title": "Qualification",
"visited": true
}
]
},
{
"title": "Quotes",
"icon": "gw-user-info",
"steps": [
{
"title": "Your Home",
"visited": true
}
]
},
{
"title": "Final Details",
"icon": "gw-insert-drive-file",
"steps": [
{
"title": "Details",
"active": true
},
{
"title": "More details"
}
],
"isError": false
},
{
"title": "Payment",
"icon": "gw-credit-card",
"steps": [
{
"title": "Construction"
},
{
"title": "Your Discounts"
},
{
"title": "Your Quote"
}
]
}
]
}
}
}
Step progress bar
A StepProgressBar
renders a progress bar with clearly marked steps.
Adding steps to step progress bar
Add an array of steps to the steps
prop. title
is required for each step.
const steps = [
{
active: true,
title: 'Your Business',
visited: false,
},
{
active: false,
title: 'Quotes',
visited: false,
},
{
active: false,
title: 'Final Details',
visited: false,
},
{
active: false,
title: 'Payment',
visited: false,
},
{
active: false,
title: 'Payment-2',
visited: false,
},
{
active: false,
title: 'Additional information',
visited: false,
},
];
<StepProgressBar steps={steps} />;
Here's the presentation metadata for this example:
{
"$schema": "../metadata.schema.json",
"jutro": "10.3.6",
"step.progress.example": {
"component": "StepProgressBar",
"id": "stepProgressId",
"type": "element",
"componentProps": {
"steps": [
{
"title": "Your Business",
"visited": false,
"active": true
},
{
"title": "Quotes",
"visited": false,
"active": false
},
{
"title": "Final Details",
"visited": false,
"active": false
},
{
"title": "Payment",
"visited": false,
"active": false
},
{
"title": "Payment-2",
"visited": false,
"active": false
},
{
"title": "Additional information",
"visited": false,
"active": false
}
],
"isVertical": false
}
}
}
Vertical step progress bar
When isVertical
prop is set to true
, the progress bar renders in vertical layout.